2015-05-08
Consider we have a typical libGDX-based game, such as the one generated with gdx-setup.jar
.
Tests directory
Before we can add JUnit tests to the core module of our game, let's first reorganize the directory structure.
The standard directory structure is:
src
- the Java sources directory
We'll expand that in order to separate test classes from production classes. The test resource files (if any) should also be separate.
src/main/java
- the Java sources directory - the equivalent of the previoussrc
directorysrc/test/java
- the Java test sources directory - where our unit test classes will residesrc/test/res
- the test resources directory - for resource files that are only used by tests
Open the core/build.gradle
and change this line:
sourceSets.main.java.srcDirs = [ "src/" ]
to:
sourceSets.main.java.srcDirs = [ "src/main/java/" ]
sourceSets.test.java.srcDirs = [ "src/test/java/" ]
sourceSets.test.resources.srcDirs = [ "src/test/res/" ]
Now create the new directories and move the content from the old src
to the new location. If you’re using some version control system, don’t forget to move the files in such a way that preserves history (e.g. git mv
or svn move
instead of mv
):
$ cd core/src
$ mkdir -p main/java test/java test/res
$ ls
com MyGdxGame.gwt.xml main test
$ git mv com *.gwt.xml main/java/
In this example above we had a com
package for the sources.
Now if you refresh the core project in Eclipse, you'll get some compilation errors. That's because the sources have moved from src
to src/main/java
, but the classpath still only contains src
. We'll ask Gradle to update the Eclipse project for us:
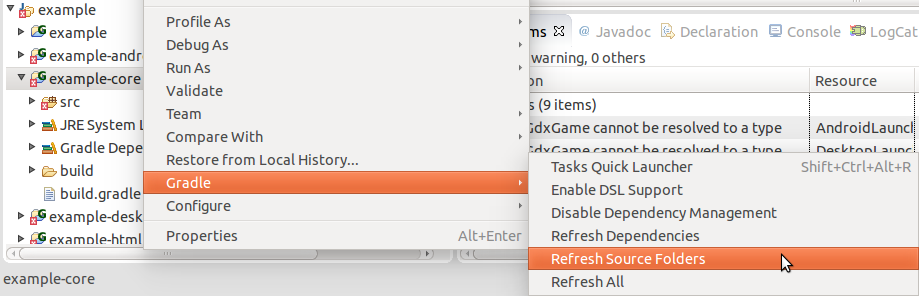
Right-click on the core project, and from the Gradle
submenu choose Refresh Source Folders
.
Adding the JUnit dependency
Open the main build.gradle
file and add the following line to the dependencies for the core
module:
testCompile "junit:junit:4.11"
So you'll end up with something like this:
project(":core") {
apply plugin: "java"
dependencies {
compile "com.badlogicgames.gdx:gdx:$gdxVersion"
testCompile "junit:junit:4.11"
}
}
Now to let Eclipse know that Gradle got new dependencies, right-click on the core
project, and from the Gradle
submenu choose Refresh Dependencies
.
That's it, now JUnit should be available in the core
.